Welcome to the beginner’s guide to demystifying Lambda expressions in Java!
If you’ve just started your journey in Java programming, understanding Lambda expressions may seem daunting at first. This article will help unravel the mystery and empower you to leverage this feature.
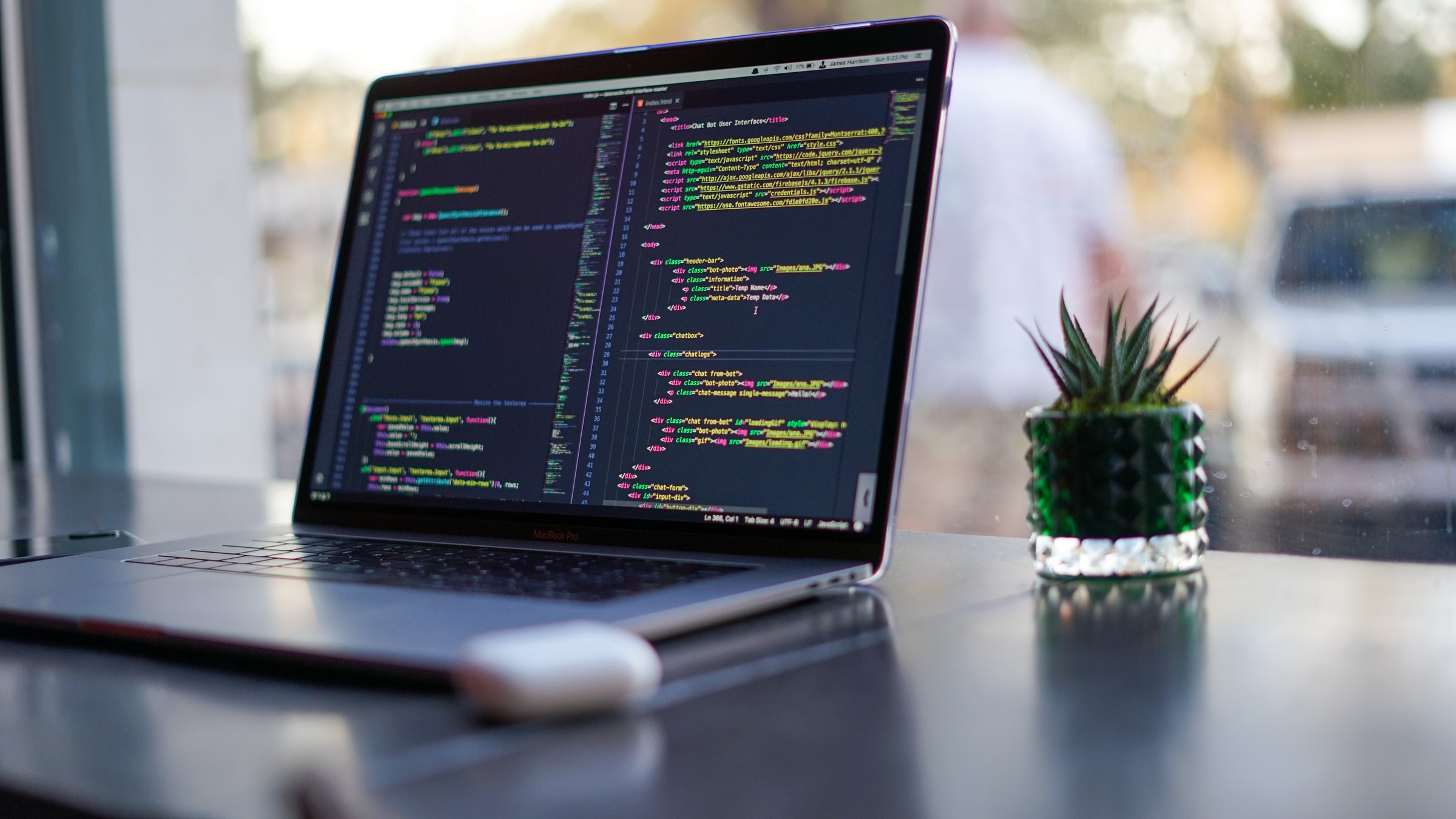
Lambda expressions were introduced in Java 8. They have revolutionized the way developers write code. Lambda provides a concise and expressive way to represent anonymous functions, making your code more readable and maintainable.
In this guide, we’ll take you step by step through the concept of Lambda expressions in Java. From understanding the syntax to practical examples, you’ll gain a solid foundation to start using Lambda expressions effectively in your programs. So, let’s dive in and unlock the potential of Lambda expressions in Java!
What are lambda expressions?
- Lambda expressions are a way to represent a block of code as an object.
- Lambda expressions provide a shorter syntax compared to traditional Java methods, making your code more concise and expressive.
- They are particularly useful when working with collections and streams, as they allow you to write compact code for filtering, mapping, and reducing data.
Syntax and structure of lambda expressions
The syntax of a lambda expression consists of three parts:
- the parameter list which specifies the types and names of the parameters,
- the arrow token -> which separates the parameters from the body,
- the body that contains the code.
Lambda expressions can be written in two forms: with or without explicit types.
- In the explicit types form, the parameter types are explicitly declared.
- In the inferred types form, the compiler infers the types from the context.Here’s a simple example of a lambda expression that adds two numbers:
// Explicit types MathOperation addition = (int a, int b) -> a + b; // Inferred types MathOperation addition = (a, b) -> a + b;
Functional Interfaces in Java
The lambda expressions concept relates to the functional interfaces.
A functional interface contains only one abstract method. It serves as a contract for lambda expressions, specifying its signature.
Functional interfaces can be annotated with the @FunctionalInterface annotation. It is optional but recommended. This annotation ensures that the interface has only one abstract method, preventing the accidental addition of new methods.
Java provides several built-in functional interfaces in the java.util.function package, such as Predicate, Consumer, and Function. These functional interfaces cover common use cases and provide a standardized way to work with lambda expressions.
A simple example of the lambda expression
To use lambda in Java, you need a functional interface that matches the signature of the lambda expression.
Once you have a functional interface, you can assign a lambda expression to a variable of that interface type.
Here’s an example that demonstrates the usage of lambda expressions. Here, the functional interface is Predicate.
List<String> names = Arrays.asList("John", "Jane", "Alice", "Bob"); // Using a lambda expression to filter names starting with "J" List<String> filteredNames = names.stream() .filter(name -> name.startsWith("J")) .collect(Collectors.toList());
In this example, the lambda expression (name -> name.startsWith(“J”)) is used as the argument to the filter method. It represents a predicate that tests if a name starts with “J”.
The resulting list contains only the names that satisfy the predicate.
Benefits of lambda expressions
Lambda expressions offer several benefits in Java development. Let’s list some of them:
- They allow you to write more concise and expressive code. By eliminating the need for anonymous inner classes, lambda expressions reduce the boilerplate code and make your code easier to read and understand.
- Lambda expressions enable you to write more flexible and reusable code. By treating functionality as a method argument or code as data, you can pass behavior to methods and classes, making your code more modular and adaptable.
- Lambda expressions enhance the power of functional programming in Java. They enable you to take advantage of functional programming concepts such as immutability, higher-order functions, and pure functions. This leads to code that is more robust, testable, and maintainable.
Two other examples of lambda expressions in Java
To further illustrate the usage of lambda expressions in Java, let’s look at a few examples. We’ll start with a simple example of sorting a list of integers in ascending order:
List<Integer> numbers = Arrays.asList(5, 2, 8, 1, 9); // Sorting in ascending order using lambda expressions numbers.sort((a, b) -> a - b);
In this example, the lambda expression (a, b) -> a – b is used as the argument to the sort method. The resulting list is sorted in ascending order.
Another example: let’s calculate the sum of all even numbers in a list:
List<Integer> numbers2 = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10); // Calculating the sum of even numbers using lambda expressions int sum = numbers2.stream() .filter(number -> number % 2 == 0) .mapToInt(number -> number) .sum();
In this example, the lambda expression
number -> number % 2 == 0
is used as the argument for the filter method.
The resulting sum contains the sum of all even numbers in the list.
Common use cases for lambda expressions
Lambda expressions are widely used in Java for various purposes. Some common use cases include:
- Collection manipulation: Lambda expressions are used to filter, map, and reduce collections. They provide a concise way to perform complex operations on collections, such as finding the highest value, calculating the average, or grouping elements by a certain criterion.
- Event handling: Lambda expressions can handle events in graphical user interfaces. They allow you to define the behavior of an event listener in a compact and readable way, making your code more maintainable and easier to understand.
- Multithreading: Lambda expressions can be used with the java.util.concurrent package to simplify the creation of threads and execution of tasks. They provide a convenient way to express concurrent behavior.
- Functional programming: Lambda expressions has in focus on functional programming concepts in Java. They allow you to write code that is more declarative, and immutable.
Lambda expressions vs anonymous inner classes
Lambda expressions are often compared to anonymous inner classes, as they serve a similar purpose of representing anonymous functions. However, lambda expressions offer several advantages over anonymous inner classes. Let’s check them:
- Lambda expressions provide a more concise syntax compared to anonymous inner classes. They eliminate the need for explicit declaration of types and method names, making your code more readable and less verbose.
- Lambda expressions are more efficient in terms of performance. They don’t create a new class file for each anonymous function.
- Lambda expressions are more flexible and expressive than anonymous inner classes. They allow you to pass behavior as a parameter to methods and classes.
Conclusions
Lambda expressions are a powerful feature of Java that can significantly improve the readability and maintainability of your code.
By leveraging lambda expressions, you can write more concise and expressive code, embrace functional programming concepts, and enhance the overall quality of your Java programs.
Keep coding…
💻 For more Java Guides, check out Code in Java